How to sort an array alphabetically in javascript
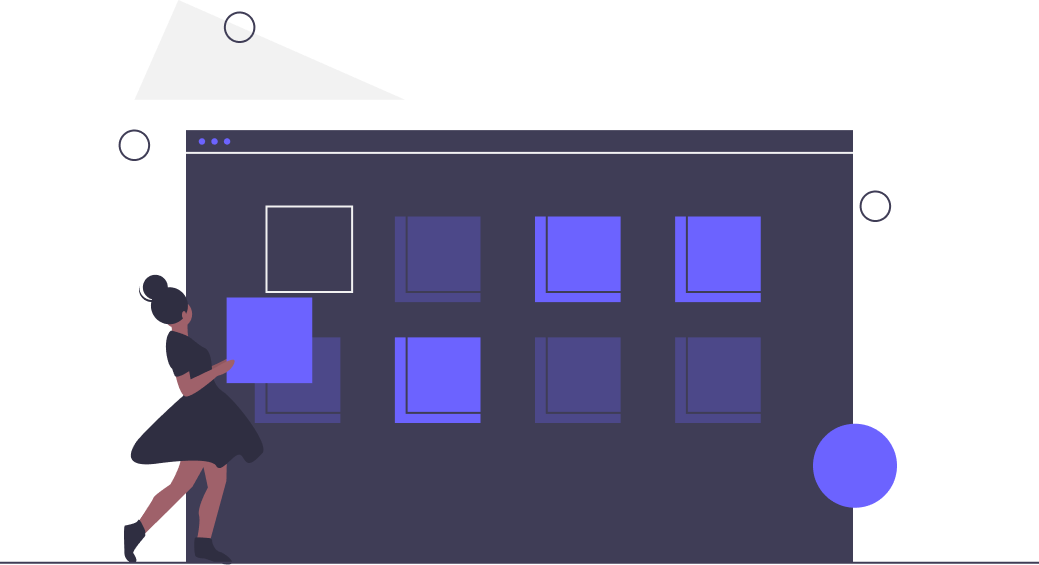
To sort an array alphabetically in javascript you can use the sort function.
To Sort a-z.
["blue", "red", "green", "yellow", "purple"].sort();
//outputs ["blue", "green", "purple", "red", "yellow"]
This works well but what if there is a mix between lower case and uppercase? eg
["blue", "Green", "red", "green", "yellow", "purple", "Silver"].sort();
//outputs ["Green", "Silver", "blue", "green", "purple", "red", "yellow"]
Despite what you might expect the sort method sorts alphabetically but has the capitalised letters words before the lowercase letters.
A more robust solution which will also handle non standard letters such as é is to use the localeCompare.
So now
["blue", "Green", "red", "green", "yellow", "purple", "Silver"].sort((a, b) =>
a.localeCompare(b)
);
//outputs ["blue", "green", "Green", "purple", "red", "Silver", "yellow"]
As discussed in our article on sorting numerically there is also another syntax which you might come across which can be a bit cleaner and reusable.
const sortAtoZ = (a, b) => a.localeCompare(b);
const sortZtoA = (a, b) => b.localeCompare(a);
This will allow us to pass in our function directly into the sort.
eg
console.log(
["blue", "Green", "red", "green", "yellow", "purple", "Silver"].sort(sortAtoZ)
);
//outputs ["blue", "green", "Green", "purple", "red", "Silver", "yellow"]
console.log(
["blue", "Green", "red", "green", "yellow", "purple", "Silver"].sort(sortZtoA)
);
//outputs ["yellow", "Silver", "red", "purple", "Green", "green", "blue"]
I find this the best solution because we are able to test this function and reuse it with confidence across our codebase, also by giving a descriptive name to the function we help prevent getting our orders mixed up.