Create a Cricket Points Table in Javascript - Part 3
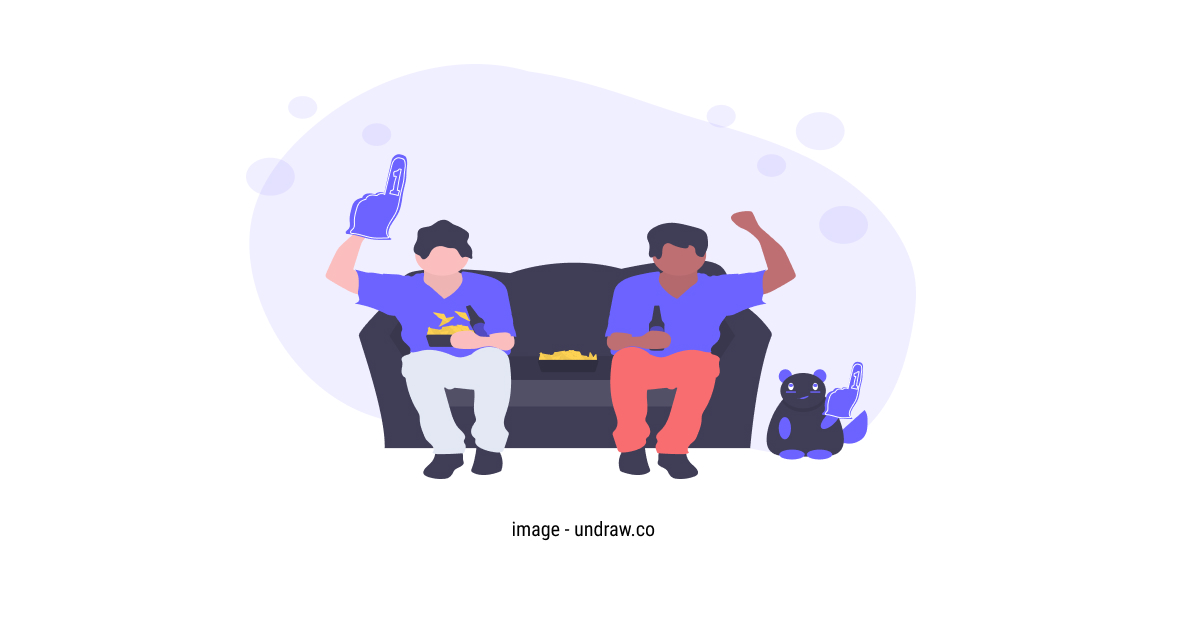
In this tutorial we are going to create functions to calculate the total number of wins, losses, no results and points a team has for the tournament.
Jump To Section
- Overview
- Calculate Totals
- Calculate Total Won
- Calculate Total Lost
- Calculate NO Result
- Calculate Points
Overview
Click on the following links to read part1 or part2 of the series.
In this tutorial we are going to build on the functions we created in our last post (teamWon,teamLost etc) and create functions to calculate the total number of wins, losses, no results and points a team has for the tournament.
Calculate Totals
We are going to create a reusable function where we can pass in the below params.
- config - An Object which will determine the points allocated for a win in the comp eg
{ pts4Win: 2, pts4NR: 1 }
- matches - Our matches array
- team - The Team eg IND (in this post we will focus on one team but in future posts we will loop over all teams)
- stat - This function will be able to be used for WON,LOST,NORESULT,POINTS
export const const cricketTeamTotalCalc = ({
config,
matches,
team,
stat
}) => {}
There are a few ways we can do this but in this case we are going to use a switch statement
switch (stat) {
case WON:
return; /* calc WON */
case LOST:
return; /* calc LOST */
case NORESULT:
return; /* calc NORESULT */
case POINTS:
return; /* calc POINTS */
default:
return;
}
Calculate Total Won
We will tally up the amount of wins the team has had when they are team 1 and team 2
case WON:
return (
teamWon({
matches,
teamNum: TEAM1,
team,
stat: "Ru"
}) +
teamWon({
matches,
teamNum: TEAM2,
team,
stat: "Ru"
}) +
teamWonSuperOver({
matches,
teamNum: TEAM1,
team,
stat: "SupOvrR"
}) +
teamWonSuperOver({
matches,
teamNum: TEAM2,
team,
stat: "SupOvrR"
})
);
Tests
const config = { pts4Win: 2, pts4NR: 1 };
expect(
cricketTeamTotalCalc({
config,
matches,
team: "SA",
stat: WON,
})
).toBe(3);
Calculate Total Lost
We will tally up the amount of losses the team has had when they are team 1 and team 2
case LOST:
return (
teamLost({
matches,
teamNum: TEAM1,
team,
stat: "Ru"
}) +
teamLost({
matches,
teamNum: TEAM2,
team,
stat: "Ru"
}) +
teamLostSuperOver({
matches,
teamNum: TEAM1,
team,
stat: "SupOvrR"
}) +
teamLostSuperOver({
matches,
teamNum: TEAM2,
team,
stat: "SupOvrR"
})
);
Tests
const config = { pts4Win: 2, pts4NR: 1 };
expect(
cricketTeamTotalCalc({
config,
matches,
team: "SA",
stat: LOST,
})
).toBe(5);
Calculate NO Result
We will tally up the amount of No Results the team has had when they are team 1 and team 2
case NORESULT:
return (
teamNoResult({
matches,
teamNum: TEAM1,
team
}) +
teamNoResult({
matches,
teamNum: TEAM2,
team
})
);
Tests
const config = { pts4Win: 2, pts4NR: 1 };
expect(
cricketTeamTotalCalc({
config,
matches,
team: "SA",
stat: NORESULT,
})
).toBe(1);
Calculate Points
We will tally up the wins and multiply them by the number passed in our config to work out how many points the team has.
case POINTS:
return (
(teamWon({
matches,
teamNum: TEAM1,
team,
stat: "Ru"
}) +
teamWon({
matches,
teamNum: TEAM2,
team,
stat: "Ru"
}) +
teamWonSuperOver({
matches,
teamNum: TEAM1,
team,
stat: "SupOvrR"
}) +
teamWonSuperOver({
matches,
teamNum: TEAM2,
team,
stat: "SupOvrR"
})) *
pts4Win +
+(
teamNoResult({
matches,
teamNum: TEAM1,
team
}) +
teamNoResult({
matches,
teamNum: TEAM2,
team
})
) *
pts4NR
);
Tests
const config = { pts4Win: 2, pts4NR: 1 };
expect(
cricketTeamTotalCalc({
config,
matches,
team: "SA",
stat: POINTS,
})
).toBe(7);
....
In this lesson we have started to create the building blocks required for our points table application and in Part 4 we are going to create a function to calculate overs and Net Run Rate.
To see the change in full head over to the github repo