How to filter an array in javascript
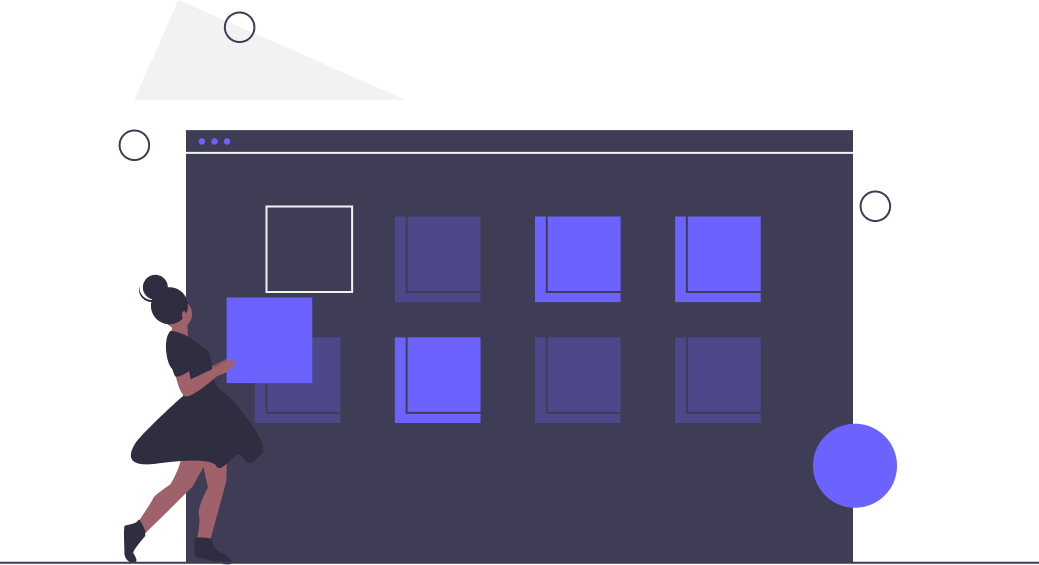
To filter an array in Javascript we can pass in a condition to the built in filter function.
For example in the below array.
const myArray = [
{ id: 111, name: "John", age: 29 },
{ id: 112, name: "Sarah", age: 25 },
{ id: 122, name: "Kate", age: 22 },
{ id: 123, name: "Tom", age: 21 },
{ id: 125, name: "Emma", age: 24 },
];
we can return results where the age is less than 22
myArray.filter((user) => user.age < 22);
Greater than 22
myArray.filter((user) => user.age > 22);
And we can take advantage of chaining to add multiple filters eg greater than 22 and less than 29.
myArray.filter((user) => user.age > 22).filter((user) => user.age < 29);
Note: in the examples, user refers to the current item in the array, you can use what ever you want here, its best to use something that you will easily understand when you come back to the code later.