How to sort an array numerically in javascript
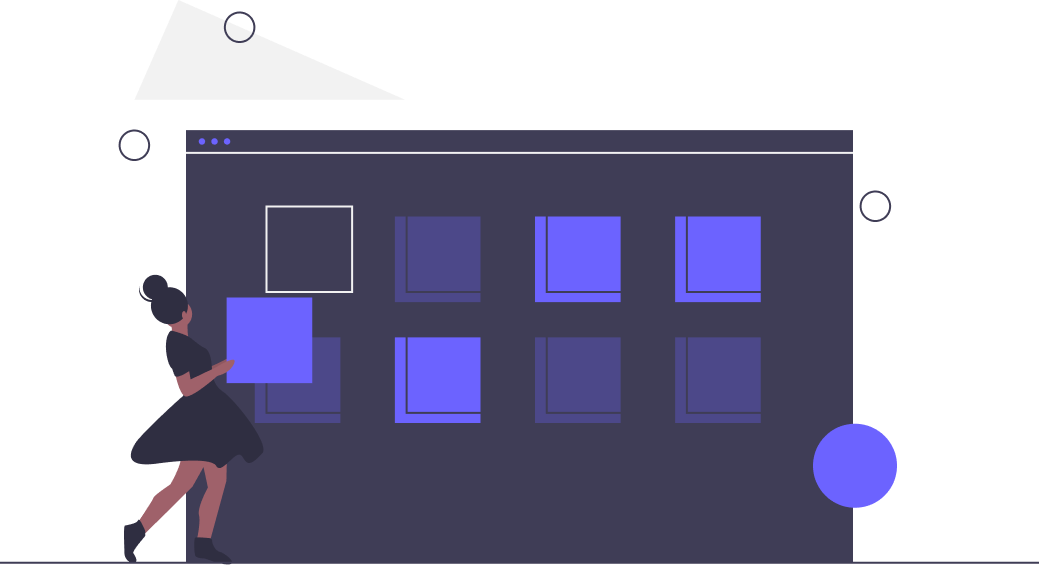
To sort an array numerically in javascript you pass a Compare Function to the array you want to sort.
Jump To Section
Sort Array in ascending order javascript
To Sort in ascending order (lowest to highest)
[1, 5, 3, 9, 2, 11].sort((a, b) => a - b);
//outputs [1, 2, 3, 5, 9, 11]
Sort Array in descending order javascript
To Sort in descending order (highest to lowest) swap the a and the b around
[1, 5, 3, 9, 2, 11].sort((a, b) => b - a);
//outputs [11, 9, 5, 3, 2, 1]
So far so good but what about if our numbers are coming through as a string? The built in compare function will handle this for us.
["1", "55", "3", "9", "2", "11"].sort((a, b) => a - b);
//outputs ["1", "2", "3", "9", "11", "55"]
There is also another syntax which you might come across which can be a bit cleaner and reusable.
const highestToLowest = (a, b) => b - a;
const lowestToLowest = (a, b) => a - b;
This will allow us to pass in our function directly into the sort.
eg
console.log([1, 5, 3, 9, 2, 11].sort(highestToLowest));
//outputs [11, 9, 5, 3, 2, 1]
console.log([1, 5, 3, 9, 2, 11].sort(lowestToLowest));
//outputs [1, 2, 3, 5, 9, 11]
I find this the best solution because we are able to test this function and reuse it with confidence across our codebase, also by giving a descriptive name to the function we help prevent getting our ascending and descending orders mixed up.