Create a Cricket Points Table in Javascript - Part 5
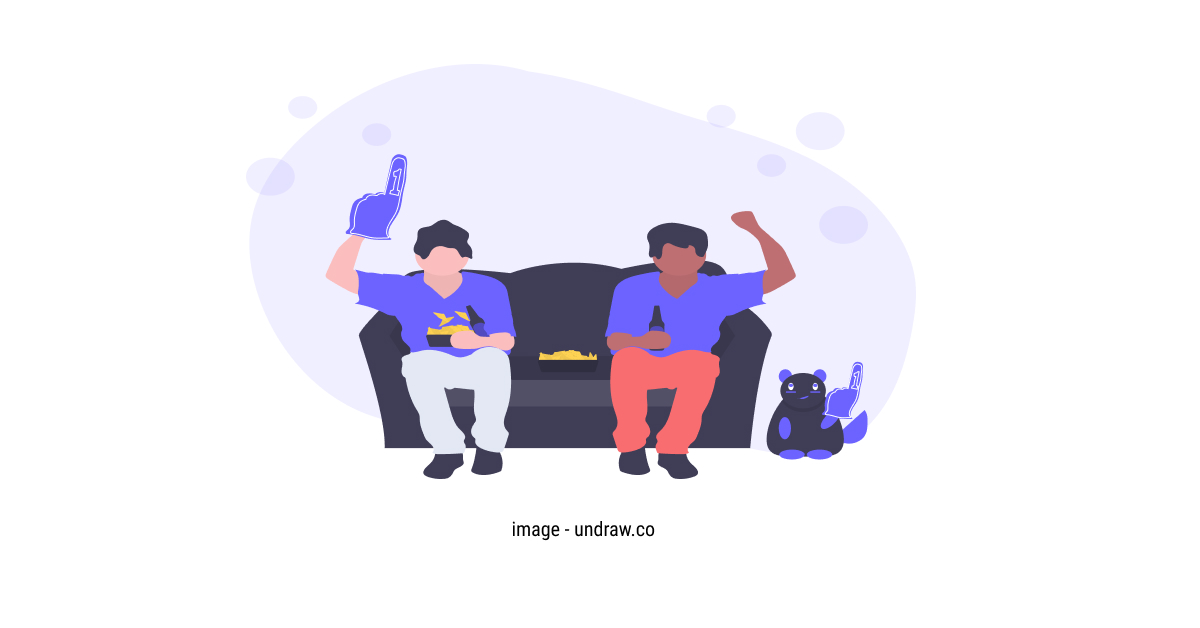
Overview
Click on the following links to read part1, part2, part3 or part4 of the series.
In this tutorial we are going to complete our course by creating an object with the points table data for all the teams in the competition then display it in a html table with react.
Get All the Teams in the competition.
To get all the teams in the competition we are going to use the matches
data we have been using throughout the course and get list on unique teams. (Because we know the teams we could simply output an array however this will function will allow us to easily get the list on any future tournaments as well).
To do this we will map
the matches array to return t1 and t2 for each match.
eg matches.map(match => [match.t1, match.t2])
will output [[["ENG", "SA"], ["WI", "PAK"]]
(for all the matches in the tournament)
Then we can use the .flat()
method to turn our array of arrays into a single array so our data will now look like this [["ENG", "SA", "WI"]]
We will then remove any blanks from the dataset and sort in alphabetical order [["AFG", "AFG", "AFG"]]
(each team will appear multiple times one for every match they are in).
Now if we wrap our arr in set
we will get a unique list of teams ...new Set()
eg ["AFG", "AUS", "BAN", "ENG"]
Full Example
const teamsInMatches = (matches) => [
...new Set(
matches
.map((match) => [match.t1, match.t2])
.flat()
.filter((item) => item !== "")
.sort()
),
];
Tests
it("teamsInMatches", () => {
expect(teamsInMatches(matches)).toEqual([
"AFG",
"AUS",
"BAN",
"ENG",
"IND",
"NZ",
"PAK",
"SA",
"SL",
"WI",
]);
});
Store the points table data
In the previous post we created a function called cricketPointsTableStats
which returned all the data for a team for the points table (played, won, lost etc), now we will combine this function with our teams function above to return the data for all teams.
import { cricketPointsTableStats } from "./cricketPointsTableStats";
const pointsTableObj = ({ teams, matches, config }) => {
return teams.reduce((obj, team) => {
return {
...obj,
[team]: cricketPointsTableStats({
matches,
team,
config,
}),
};
}, {});
};
Which will output
{
"IND":{ /* IND Data */},
"ENG":{ /* ENG Data */}
/* all other teams */
}
Sort The Points Table
To sort the points table we will sort by three conditions.
- Sort by points.
- If points are equal sort by matches won.
- If the wins are equal sort by net run rate.
const sortPointsTable = (arr) =>
arr.sort((a, b) => {
if (a.pts === b.pts && a.won === b.won) {
return b.netrr - a.netrr;
} else if (a.pts === b.pts) {
return b.netrr - a.netrr;
} else {
return b.pts - a.pts;
}
});
Render data in react
All code so far in this course has no dependency on React JS
however we setup a create-react-app
project so are now going to use it to display our data.
In our src/index.js
file we will add in get our pointsTableObj
we created earlier and use our sort
method from above to get our data in an array.
const pointsTableData = pointsTableObj({
teams: teamsInMatches(matches),
matches,
config: tournamentConfig,
});
const sortedPointsTable = sortPointsTable(
Object.keys(pointsTableData).map((key) => pointsTableData[key])
);
output (first result only)
{
team: "IND",
played: 9,
won: 7,
lost: 1,
noresult: 1,
runsFor: 2260,
oversFor: 381,
runsAgainst: 1998,
oversAgainst: 390,
netrr: "0.809",
pts: 15
}
Then we will output the data in a html table. (If you refer to the code you will also see some initial css in here as well but I have left it out of the blog post feel free to improve the layout of the page!)
{
sortedPointsTable.map((team) => (
<tr key={team.team}>
<td>{team.team}</td>
<td align="right">{team.played}</td>
<td align="right">{team.won}</td>
<td align="right">{team.lost}</td>
<td align="right">{team.netrr}</td>
<td align="right">{team.pts}</td>
</tr>
));
}
To follow the code so head over to codesandbox.
There are many ways to create a cricket points table in JavaScript. I really hope I was able to provide some value to you in this course. Please share with your friends and colleagues if it did and be sure to follow and leave a comment on social media if you have any questions, suggestions, improvements.
Have a great day!